Frontend Developer: Do Repeat Yourself
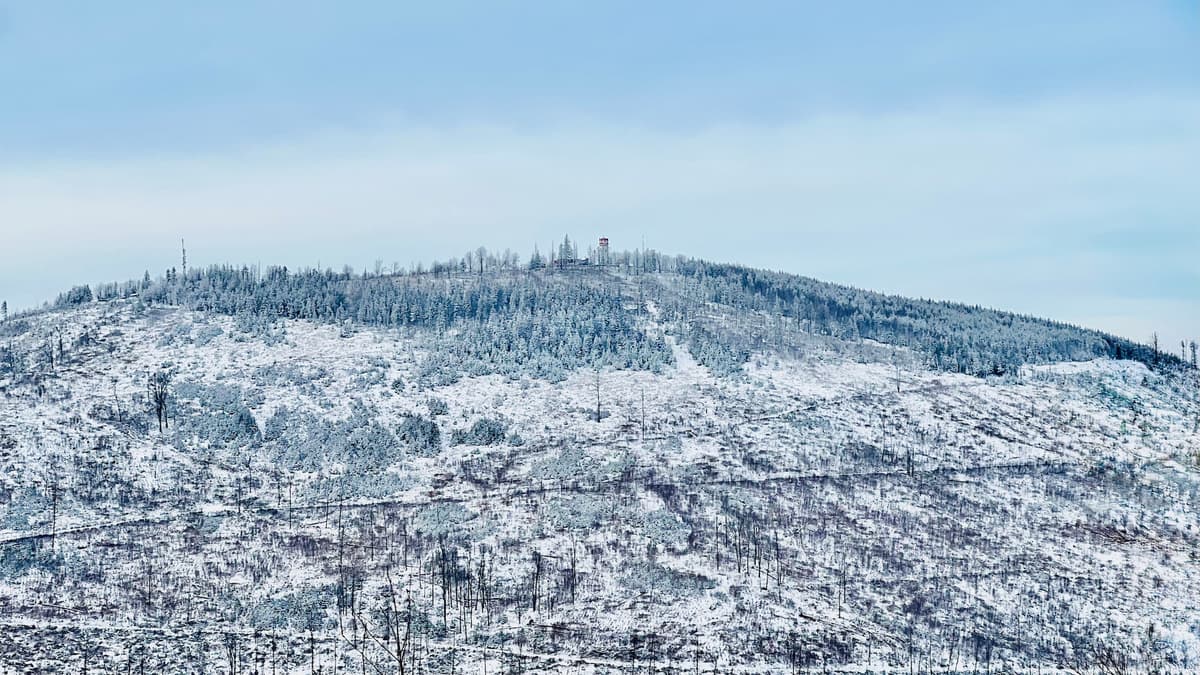
import UsersTable from ’../assets/UsersTable.svg’
Don’t Repeat Yourself, also known as DRY, is a foundational principle in software development, often learned early in a developer’s journey. At first glance it sounds simple and straightforward. But can it be misapplied? Let’s explore it from the frontend perspective.
Don’t Confuse Simple With Easy#
The main problem with the DRY rule is that it appears simple, straightforward, and universal. It’s almost a truism we can imagine applying to many areas of life. In the end, how can one misunderstand the Don’t Repeat Yourself phrase, right?
I believe this perception of simplicity is precisely why the principle is often easy to misunderstood, especially by developers early in their careers. The reality is:
What’s easy isn’t always simple, and what’s simple isn’t always easy.
DRY is a perfect example of this – a rule so obvious that we assume it’s easy to use. While code repetition is generally considered poor practice, blindly applying any paradigm, design pattern, or rule without careful thought inevitably creates more problems than benefits.
Let’s discuss some challenges that may arise when applying the DRY principle too eagerly:
- Premature Abstraction Problem
- Misunderstanding Components Purpose
- Rushing to Generalize
- Forgetting The Why
Premature Abstraction Problem#
A premature abstraction occurs when we introduce it before we have enough context to make a fully conscious decision. This can happen at several levels:
- In code
When we try to split code logically before we fully understand the problem or consider edge cases that need time to emerge. - In architecture
When we split an application into multiple services based only on assumptions or predictions, without a clear understanding of scope. - In general approaches
When we prebuild a design system library (or any other “toolbox package”) without solid knowledge of how users will actually use it.
While the list could go on, I want to focus on the frontend perspective, as it seems to be specifically prone to this issue. However, before diving in, it’s important to note that we should generally avoid abstracting things too early in the development process.
Misunderstanding Components Purpose#
Thinking in components is an inherent part of modern frontend development. Since we do this constantly, it’s tempting to identify potentially repetitive parts too early. Moreover, since frontend engineers’ work is deeply connected to visual aspects, we often tend to view things solely from a visual perspective.
Using components just to avoid repeating HTML/CSS elements works perfectly at the atomic level. However, when developing more sophisticated structures (like molecules and organisms), the situation becomes more complex.
In these cases, it’s easy to miss the point where trying to maintain a generic approach just to avoid code repetition actually makes the code more complex than necessary.
Let’s discuss this through a real-world scenario that happened to me.
The Table of Users#
Imagine a table to display system’s users, showing their email, name, privileges, and available actions. Simple enough.
After the table is built and available under the /users
tab, a new requirement emerges: now we need to present all users during project creation as well.
What do we do? We reuse our existing component. That’s what components are for, right?
Then the design team informs us that we don’t need actions during the setup process.
Easy – we’ll just add a boolean property to control whether we render the actions or not.
A week goes by and our PM says:
“Let’s show only the most relevant privileges during project creation, but keep all of them in the users tab. Oh, and can we highlight the most recently added user, but only during project creation? That shouldn’t be a lot of work, right?”
I think you can see where this is going. Our initial decision to reuse the table from the very beginning in both places led to a maintenance nightmare.
We ended up building a complex interface just to manipulate this table as if it were part of a design system – which it isn’t. We’re forcing one component to behave like two (or more!). That’s the real problem.
So, For What Components Are For#
Reflecting on the example above, does this mean we should have created two separate tables as soon as we introduced the second use case? No. Should we have done it after the first change? I don’t know, maybe.
The point I aim to make is that:
The fact that two components look or behave similarly (or even the same) doesn’t automatically make them good candidates for abstraction.
While components serve many purposes, and preventing repetition is definitely one of them, it’s worth acknowledging that there is much more behind them.
Components help us think about and build in more modular ways with looser coupling. They also allow us to separate concerns, clarify ownership, and many more.
Perceiving components only from a visual perspective not only often leads to unnecessary complexity, but also rob us from leveraging the other benefits of modularity.
Rushing To Generalize#
Another common problem is being too eager to extract code, sometimes starting with the thought “oh, this will surely be reused – let’s make it generic.”
While it’s always good to consider potential scenarios, it’s much better to apply the DRY principle when we have a genuine need for it.
Unfortunately, there’s no rule of thumb for when to introduce abstraction, I personally prefer to have real-world examples to identify common ground. Building similar things multiple times not only helps you rethink the problem better but also helps you understand which parts are good candidates for extraction and which aren’t.
Of course, this is highly subjective and varies based on what we’re trying to abstract. Generally, it’s better to extract code only when you already know how it will be reused – or at least have a clear plan for its reuse.
Remember, principles like KISS (Keep It Simple, Stupid) and YAGNI (You Aren’t Gonna Need It) serve as essential guardrails against over-abstraction. When you’re writing code for hypothetical scenarios that may never materialize, you’re likely violating YAGNI – and making your life harder in the process.
Forgetting The Why#
The DRY principle can be applied at many levels. We can think of reusing simple functions, hooks, components, parts of styles, or even building our own frameworks. Every situation is different, and there’s no point in comparing all possible cases as that would be highly subjective.
Every situation is different, and we should always consider the “why” behind it. When considering making a piece of code reusable, it’s worth asking:
- Is this making the code more readable?
- Is this improving code performance?
- Will this change improve or diminish developer experience?
- Is there a specific reason to introduce this now, or could it wait?
- Is this the right thing to abstract?
These questions should help you make a more informed decision about abstraction.
To DRY or not to DRY#
Let’s clarify one thing: “Don’t Repeat Yourself” is essentially a good piece of advice that leads to many benefits. However, we must be cautious about when and how we apply it.
As Frontend developers, we tend to think visually, but there are many more factors to consider when deciding if something is a good candidate for abstraction.
While there’s no one-size-fits-all solution for approaching these decisions, being mindful about it is already a good start.
And finally let’s keep in mind that we can always remove abstractions later – not just introduce them.